Attributes in Swift @available
Attributes in Swift
In Swift Attributes are used to provide some kind of additional information to a declaration or a type. For example if I want a function to be just available for iOS 12 version onwards. The Swift compiler will give warnings in such cases and provides suggestion to fix it as shown below.
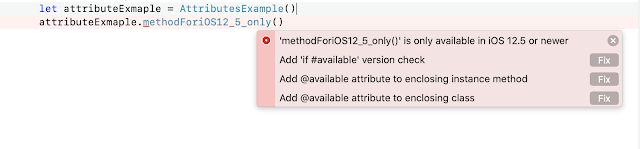
You can download the example for it from GitHub
How do we declare an attribute?
Attribute declaration starts with "@" symbol and can also arguments which is enclosed in parentheses. Arguments specify some more information on how it applies to the existing attribute declaration.
@ attributename(attributearguments)
@available(iOS,introduced: 12.5)
func methodForiOS12_5_only() {
}
Types of Attributes in Swift
There are two kinds of attributes in swift - Declaration Attributes and Type Attributes.
Declaration Attributes
As the name suggests , these can only be applied to declarations.available
discardableResult
dynamicMemberLookup
GKInspectable
inlinable
nonobjc
NSApplicationMain
NSCopying
NSCopying
objc
objcMembers
requires_stored_property_inits
testable
UIApplicationMain
usableFromInline
warn_unqualified_access
Declaration Attributes used by InterfaceBuilder
IBAction
IBOutlet
IBDesignable
IBInspectable
Type Attributes
As the name suggests it can be applied to types only.
autoclosure
convention
escaping
Details on Declaration Attribute available
Below are the list of arguments and the details to help understand the available attribute.
You can also find it's implementation once you download the project from GitHub .
@Available Attribute
This declaration attribute always appears in a list of two or more arguments. It always beings with a platform or argument.
Class : AvailableAttributeExample.swift
Implemented in Class : ViewController . availableAttributeExample
Attribute Name | Argument | Argument Details |
available | ||
Platform or langauge | This is the required argument and rest of the arguments can appear after that. Possible values are listed below. iOS , iOSApplicationExtension , macOS , macOSApplicationExtension , watchOS , watchOSApplicationExtension , tvOS , tvOSApplicationExtension , swift |
|
unavailable | It specifies the platform cannot be used on the specified platform. For first argument as swift this is not applicable |
|
introduced | The first version of language or platform in which the declaration was introduced. format: introduced: <version> <version> is three positive integers separated by a dot. |
|
deprecated | The first version of language or platform from which the declaration was deprecated. format: deprecated: <version> <version> is optional , if we wan’t to specify the declaration is deprecated for all version , skip the “version” as well as “:” |
|
obsoleted | The first version of language or platform from which it has became obsolete and cannot be used. format: obsoleted: <version> |
|
message | Provides textual message to be displayed by the compiler when emitting an error or warning. format: message: <message> <message> is a string |
|
renamed | It is usually used in conjunction with unavailable argument to specify the name of the declaration is change between releases of framework and library. |
@Available Argument - Introduced
@available(iOS,introduced: 13.5)
func methodAvailableFrom13_5() {
}
The above method is available for iOS platform and is introduced from version 13.5. As the current target version is 13.2 it will throw error.

@Available Argument - Deprecated
@available(iOS,deprecated,message: "This method has been deprecated , avoid using this method")
func deprecatedMehod() {
The above method is available for iOS platform but it obsolete and hence no longer available. Xcode will emit an error.

@Available Argument - Renamed
@available(iOS,unavailable,renamed: "formatNameWithSeperator" )
func formatName(firstName:String , lastName:String) -> String{
return "\(firstName) , \(lastName)"
}
func formatNameWithSeperator( firstName:String , lastName:String , seperator:String) -> String {
return "\(firstName) \(seperator) \(lastName)"
}
"formName" function is now unavailable as well we have mentioned that it has been renamed to a new method "formatNameWithSeperator". As we see from the above implementation renamed argument is used in conjunction with unavailable argument.
@Discardable Attribute
This attribute is used to suppress function or method warning when it returns a value and the returned value is not used.
In below example "incrementCounterValue" returns a Int value and is not used. If @discardable is not used compiler would give warning.
@discardableResult
func incrementCounterValue(inputCountValue:inout Int) -> Int {
return inputCountValue + 1
}
Implementation does not show warning even thought the returned value is not used.
func discardableAttributeExample() {
let attr = DiscardableAttributeExample()
/*
* Method retunrs a Int Value , but since it is marked with discardableResult
* The warning of the unused variable returned by the function will be suppressed
*/
var counter = 1
attr.incrementCounterValue(inputCountValue: &counter)
}
This comment has been removed by a blog administrator.
ReplyDelete